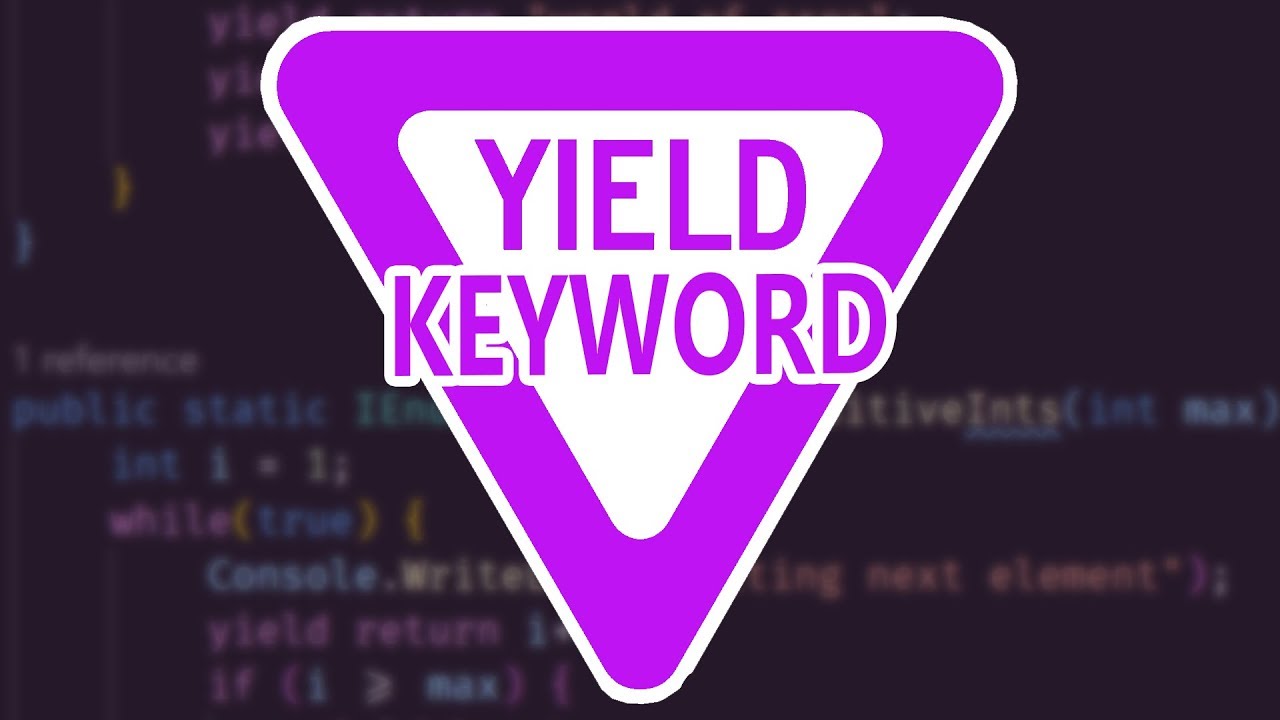
When used in a function or property which returns an `IEnumerable`, yield will provide two features. You may `return` the next value in the set or `break` out of the loop - effectively terminating the set. Values `return`ed from a `yield` keyword are composed into the enumerable set as your code evaluates. Yield statements are evaluated lazily (meaning the function generating the enumerable object is not generated completely before the first element is returned), this means that after returning with `yield` the code in the function will continue from the point it returned from including preserving the state (such as local variable values).
Using these concepts we can create a function which returns all positive integers between 1 and a max value.
```csharp
// Note: you'll need to replace the IEnumerable{int} with correct syntax as well as the ≥ symbol.
public static IEnumerable{int} PositiveInts(int max) {
int i = 1;
while(true) {
yield return i++;
if (i ≥ max) {
yield break;
}
}
}
```
* `yield return`: returns the next value in an `IEnumerable`.
* `yield break`: marks the end of the `IEnumerable`.
More information about using `yield` with some other examples is available here:
Join the World of Zero Discord Server:
0 Comments